As per the definition on Microsoft Docs: “Azure Functions is a serverless solution that allows you to write less code, maintain less infrastructure, and save on costs.”. What it means is that you when you create an Azure Function, you don’t have to worry about bootstrap code or the infrastructure, inputs and outputs of the functions are seamless as we will see and cost is practically free as under the Consumption plan 1 million executions are allowed free of cost per month per function. That is an execution approx. every 2.5 seconds, so unless you’re hitting it pretty hard, you don’t have to worry about cost.
Triggers: triggers are special types of input bindings that cause a function to execute. There is a function trigger for most commonly used Azure Services, a function can have only one trigger but it can have multiple input and output bindings. Below is the supported function triggers at the time of this writing:
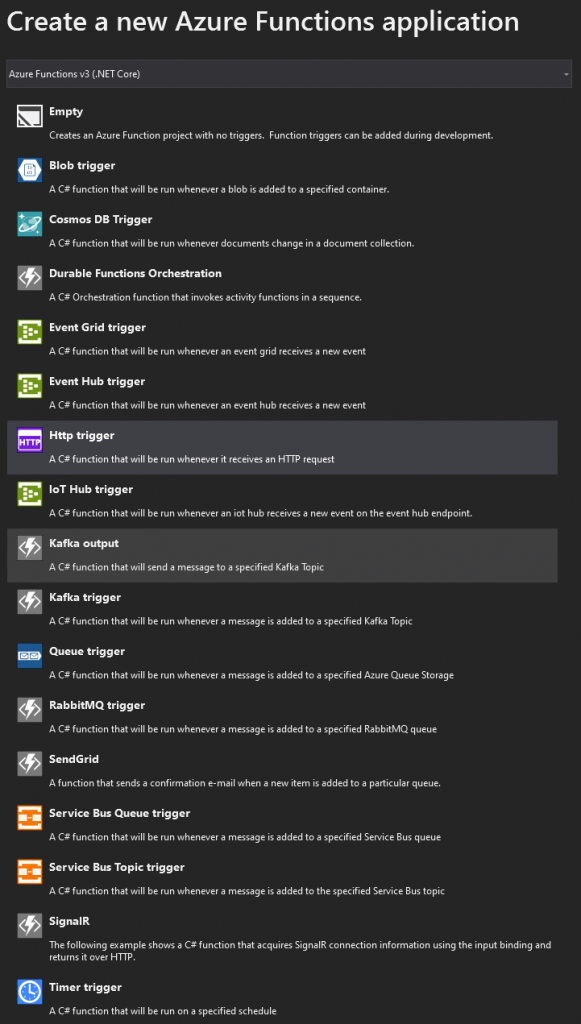
A HTTP trigger will be triggered when a HTTP request comes, Timer trigger will get triggered as per the CRON expression you defined, and similarly Queue, Topic, Event Hub/Grid and CosmosDb triggers will trigger when some new message/notification is put on the queue/topic or new entry made in the CosmosDb. A function can have only one trigger.
Let’s look bindings, there are two types of bindings that you can use with functions, input bindings (source) and output bindings (destination). Bindings are defined in JSON. A binding is configured in your function’s configuration file, which is named function.json and lives in the same folder as your function code. You must define Name, Type and Direction of the binding and for some binding types we need to define Connection as well. Look at the example below:
...
{
"name": "headshotBlob",
"type": "blob",
"path": "thumbnail-images/{filename}",
"connection": "HeadshotStorageConnection",
"direction": "in"
},
...
Notice the “direction”: “in”, it defines an input binding, In this example, we used an input binding to connect user images to be processed by our function as thumbnails, headshotBlog will be the name of your parameter in function. And then when we are done processing the image, we can use “direction”: “out” (for output) with a different blob connection to put the processed image there, in the same function. Not all binding types are supported as inputs or outputs, for more up to date details see this MS Doc.
It is bad practice to put your sensitive keys and connection strings in local.settings.json file and then commit to source code, you can put them temporarily when testing your function but don’t commit these to source code. Azure Portal Function App gives you nice place where you can put these keys and connection strings and they will be picked by your function when it runs on production.
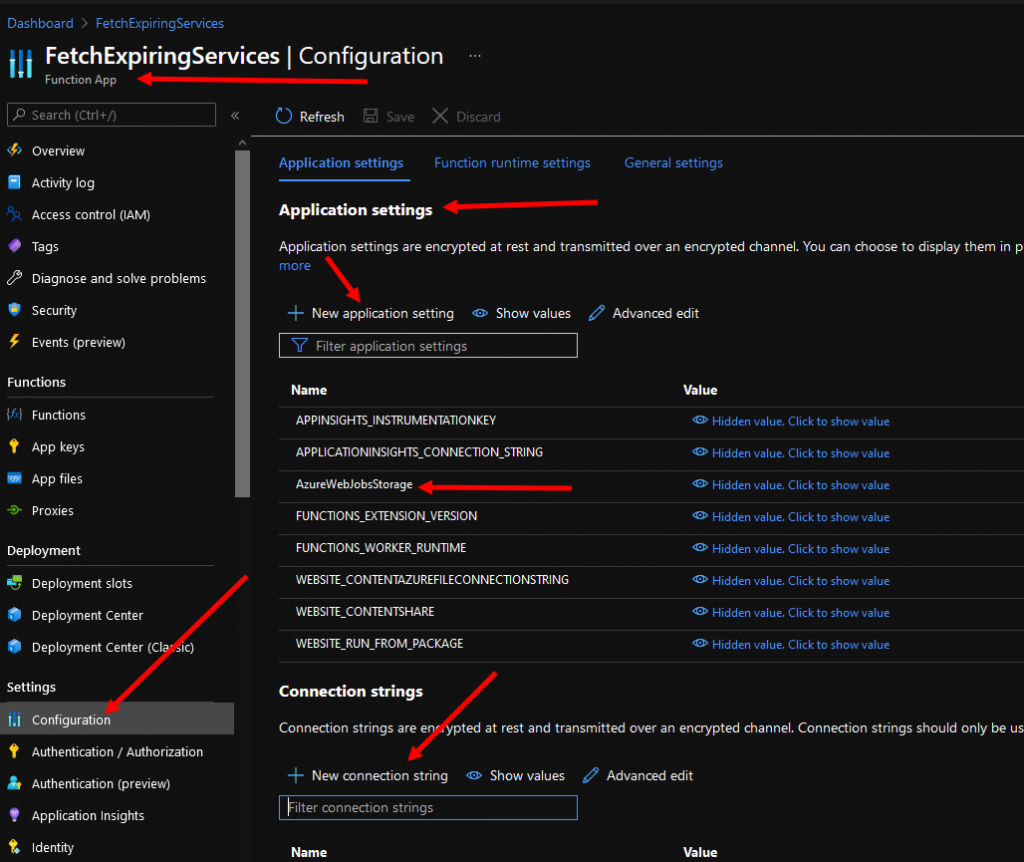
In configuration section of your Function App you can define (with same name) the application settings and connection strings as being used by your function’s ins and outs and they will be picked up by your from there. To read any application setting in your function’s code use:
...
string uri = Environment.GetEnvironmentVariable("FetchExpiringServicesFuncUriIncludingSecret");
...